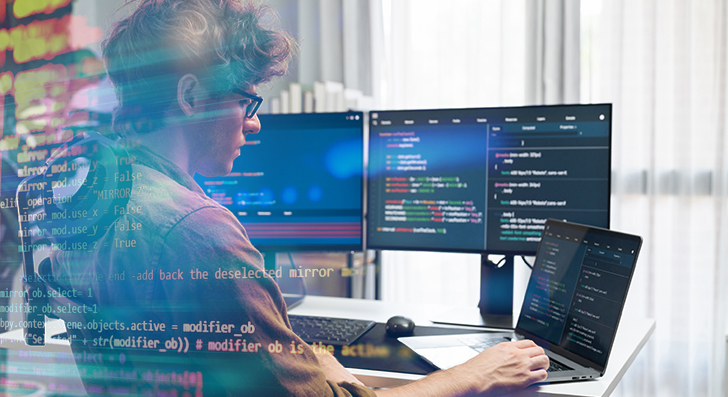
Scalability means your application can deal with growth—extra people, a lot more information, and more targeted visitors—devoid of breaking. Being a developer, developing with scalability in your mind will save time and anxiety afterwards. Listed here’s a clear and realistic guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the beginning
Scalability isn't a thing you bolt on later—it ought to be component of your respective strategy from the start. Numerous apps fall short once they improve quick mainly because the original style and design can’t deal with the additional load. As a developer, you'll want to Believe early regarding how your system will behave under pressure.
Start out by creating your architecture to get adaptable. Stay away from monolithic codebases wherever almost everything is tightly related. Rather, use modular layout or microservices. These styles break your app into more compact, unbiased parts. Each and every module or service can scale on its own with no influencing The complete method.
Also, think of your databases from working day one. Will it require to take care of one million users or perhaps a hundred? Select the suitable style—relational or NoSQL—according to how your info will increase. Plan for sharding, indexing, and backups early, even if you don’t require them but.
One more significant issue is to stay away from hardcoding assumptions. Don’t write code that only works under recent ailments. Give thought to what would materialize In the event your person foundation doubled tomorrow. Would your application crash? Would the databases slow down?
Use design and style styles that guidance scaling, like concept queues or function-pushed devices. These assistance your application tackle extra requests without the need of acquiring overloaded.
Once you Construct with scalability in mind, you're not just preparing for fulfillment—you happen to be cutting down foreseeable future problems. A well-planned system is less complicated to take care of, adapt, and increase. It’s greater to organize early than to rebuild later on.
Use the proper Databases
Deciding on the ideal databases can be a important A part of setting up scalable purposes. Not all databases are created the identical, and utilizing the Erroneous you can slow you down or even induce failures as your application grows.
Commence by comprehending your data. Could it be very structured, like rows inside a desk? If Certainly, a relational databases like PostgreSQL or MySQL is an effective fit. These are typically robust with relationships, transactions, and consistency. They also assist scaling methods like browse replicas, indexing, and partitioning to take care of far more visitors and facts.
Should your details is much more adaptable—like consumer exercise logs, solution catalogs, or files—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at handling massive volumes of unstructured or semi-structured data and may scale horizontally extra very easily.
Also, look at your study and publish patterns. Do you think you're doing a lot of reads with less writes? Use caching and skim replicas. Are you currently dealing with a major create load? Investigate databases which can manage significant write throughput, and even function-centered data storage methods like Apache Kafka (for short term facts streams).
It’s also good to think ahead. You might not have to have advanced scaling attributes now, but selecting a database that supports them signifies you received’t will need to modify afterwards.
Use indexing to hurry up queries. Avoid unneeded joins. Normalize or denormalize your knowledge determined by your obtain styles. And normally observe databases performance as you grow.
In short, the right database is determined by your app’s construction, speed needs, and how you expect it to mature. Choose time to select correctly—it’ll help save loads of hassle later on.
Enhance Code and Queries
Quickly code is key to scalability. As your application grows, each individual compact hold off adds up. Poorly written code or unoptimized queries can slow down performance and overload your procedure. That’s why it’s imperative that you Make productive logic from the start.
Start by crafting cleanse, straightforward code. Steer clear of repeating logic and take away nearly anything avoidable. Don’t select the most complicated solution if a straightforward a single functions. Keep the features short, centered, and easy to check. Use profiling equipment to seek out bottlenecks—locations where by your code normally takes as well very long to run or takes advantage of excessive memory.
Subsequent, evaluate your database queries. These normally sluggish issues down in excess of the code itself. Be certain Each and every question only asks for the data you truly require. Prevent Choose *, which fetches anything, and rather pick out distinct fields. Use indexes to hurry up lookups. And stay away from accomplishing too many joins, Specially throughout big tables.
When you discover precisely the same details getting asked for repeatedly, use caching. Keep the effects temporarily employing applications like Redis or Memcached so that you don’t really need to repeat highly-priced operations.
Also, batch your database operations if you can. In lieu of updating a row one by one, update them in teams. This cuts down on overhead and here would make your application more effective.
Remember to take a look at with significant datasets. Code and queries that work fantastic with one hundred data could crash every time they have to handle 1 million.
In a nutshell, scalable applications are rapidly applications. Keep the code limited, your queries lean, and use caching when desired. These ways help your application stay smooth and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it's to deal with far more end users plus much more website traffic. If all the things goes as a result of a person server, it will eventually immediately turn into a bottleneck. That’s wherever load balancing and caching can be found in. Both of these equipment enable maintain your app quickly, stable, and scalable.
Load balancing spreads incoming visitors throughout various servers. In place of just one server executing every one of the perform, the load balancer routes consumers to various servers based on availability. This means no one server will get overloaded. If a single server goes down, the load balancer can deliver visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing info quickly so it could be reused swiftly. When consumers request the exact same data once more—like an item webpage or a profile—you don’t really need to fetch it through the database anytime. You'll be able to provide it through the cache.
There are two prevalent sorts of caching:
1. Server-aspect caching (like Redis or Memcached) shops facts in memory for quickly obtain.
2. Shopper-side caching (like browser caching or CDN caching) outlets static information near the user.
Caching lowers database load, enhances velocity, and would make your app far more efficient.
Use caching for things that don’t improve usually. And normally ensure your cache is up-to-date when information does adjust.
In short, load balancing and caching are straightforward but impressive resources. Jointly, they help your application tackle much more people, continue to be quick, and Get well from complications. If you plan to increase, you would like each.
Use Cloud and Container Equipment
To make scalable applications, you will need instruments that let your app expand quickly. That’s where by cloud platforms and containers come in. They give you versatility, lessen set up time, and make scaling A great deal smoother.
Cloud platforms like Amazon Web Providers (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and expert services as you would like them. You don’t have to obtain components or guess upcoming potential. When traffic increases, you are able to include a lot more assets with only a few clicks or instantly employing automobile-scaling. When site visitors drops, it is possible to scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and safety instruments. You may center on constructing your app as opposed to handling infrastructure.
Containers are An additional key Software. A container offers your app and every little thing it must operate—code, libraries, configurations—into one particular unit. This can make it uncomplicated to maneuver your application among environments, out of your notebook to your cloud, with no surprises. Docker is the most popular tool for this.
Once your app uses various containers, equipment like Kubernetes enable you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single part of your respective app crashes, it restarts it instantly.
Containers also make it very easy to independent aspects of your app into services. You may update or scale elements independently, which is perfect for overall performance and dependability.
In brief, applying cloud and container resources usually means it is possible to scale fast, deploy simply, and recover speedily when difficulties materialize. If you'd like your application to develop devoid of limits, start out using these equipment early. They help you save time, minimize possibility, and assist you to keep centered on developing, not repairing.
Observe Every thing
In case you don’t monitor your application, you received’t know when things go Improper. Checking allows you see how your app is doing, location challenges early, and make much better choices as your application grows. It’s a vital part of creating scalable programs.
Start out by monitoring basic metrics like CPU usage, memory, disk Area, and response time. These inform you how your servers and expert services are carrying out. Equipment like Prometheus, Grafana, Datadog, or New Relic may help you accumulate and visualize this details.
Don’t just monitor your servers—keep track of your app as well. Keep watch over just how long it requires for end users to load web pages, how frequently problems come about, and wherever they come about. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Put in place alerts for significant problems. For example, if your reaction time goes earlier mentioned a Restrict or possibly a provider goes down, you must get notified quickly. This aids you resolve problems fast, often right before people even observe.
Monitoring can also be useful after you make improvements. In case you deploy a fresh function and find out a spike in errors or slowdowns, you could roll it back again prior to it causes serious hurt.
As your app grows, targeted visitors and facts boost. With out checking, you’ll skip indications of difficulties till it’s much too late. But with the best resources set up, you remain on top of things.
In a nutshell, checking will help you keep your application reliable and scalable. It’s not almost spotting failures—it’s about understanding your technique and making sure it really works well, even under pressure.
Final Ideas
Scalability isn’t only for huge providers. Even tiny applications require a robust foundation. By planning carefully, optimizing properly, and utilizing the right equipment, you can Create applications that develop efficiently without breaking under pressure. Start out small, Assume big, and Create good.